Introduction
Okay, so we have already learned about child-parent and parent-child communication in component. But there is a way in Lightning Experience to communicate across LWC, Aura Components, and even VisualForce pages!
We’re going to create a Mage Messaging App as given below, fill it with a VisualForce Page, an Aura Component, and an LWC, and then we will communicate messages across them using the Lightning Messaging Services. It’s gonna be a fun ride so be excited!
Messaging Channels work on a Publish Subscribe model, where messages are published (Like radio channels are broadcasted for everyone) and the subscribers listen for the messages and receive them (like radio owners tuning their radios to subscribe to channels). But enough blah-blah, let’s get to action:
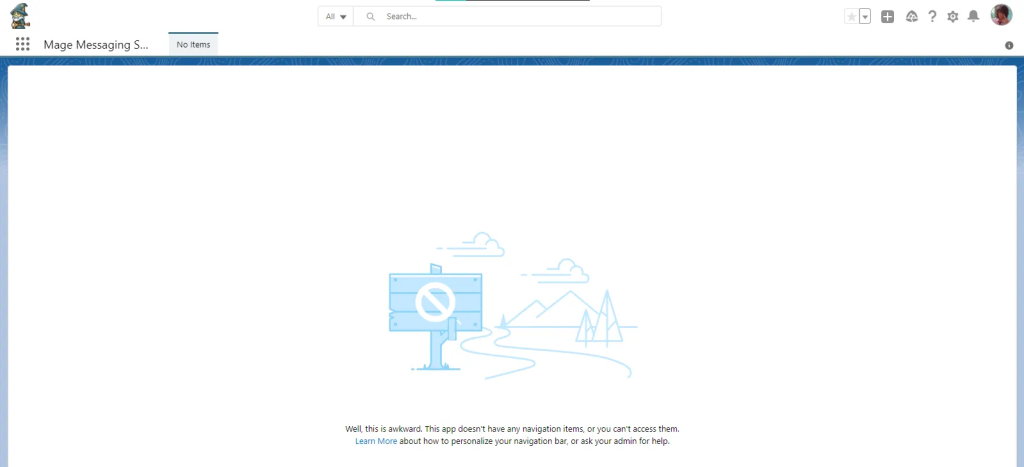
Tutorial:
1. Create a Messaging Channel
To create a channel you have to create an SFDC Project, like you do for an LWC and in ../force-app/main/default you have to create a directory named messageChannels, and within it, create a message channel with the naming convention yourChannelName.messageChannel-meta.xml as I name mine mageMessageChannel
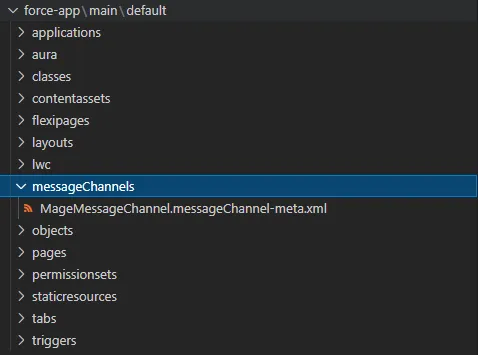
The XML for the syntax is self explanatory so I will leave it up to you guys to understand it, deploy it.
<!-- MageMessageChannel.messageChannel-meta.xml -->
<?xml version="1.0" encoding="UTF-8"?>
<LightningMessageChannel xmlns="http://soap.sforce.com/2006/04/metadata">
<masterLabel>MageMessage</masterLabel>
<isExposed>true</isExposed>
<description>Demonstrating the magic of LMS</description>
<lightningMessageFields>
<fieldName>currentMessage</fieldName>
<description>This is the only field that we are going to declare, but you can
declare as many as you want.</description>
</lightningMessageFields>
</LightningMessageChannel>
2. Create a Messaging Channel
1. Create an LWC with publish and subscribe functionality as follows. (Don’t worry the code just looks intimidating but is simple)
//mageMessageLwc.js
import { LightningElement, wire } from 'lwc';
import mageIcon from '@salesforce/resourceUrl/mageIcon'; //The cute icon for the lightning-card
// Import message service features required for publishing and the message channel
import { subscribe,
unsubscribe,
APPLICATION_SCOPE,
publish,
MessageContext } from 'lightning/messageService';
import mageMessageChannel from '@salesforce/messageChannel/MageMessageChannel__c';
export default class MageMessageLwc extends LightningElement {
subscription = null;
mageIcon = mageIcon;
lwcMessage = "This message comes from LWC!";
currentMessage;
@wire(MessageContext)
messageContext;
/* 👇🏻 Code To Publish the Message 👇🏻 */
handleSend() {
const payload = { currentMessage: this.lwcMessage };
publish(this.messageContext, mageMessageChannel, payload);
console.log("Message published");
}
/* 👇🏻 Code To Subscribe to the MessageChannel 👇🏻 */
subscribeToMessageChannel() {
if (!this.subscription) {
this.subscription = subscribe(
this.messageContext,
mageMessageChannel,
(message) => this.handleMessage(message),
{ scope: APPLICATION_SCOPE }
);
}
}
unsubscribeToMessageChannel() {
unsubscribe(this.subscription);
this.subscription = null;
}
// Handler for message received by component
handleMessage(message) {
console.log("Message Came", message);
this.currentMessage = message.currentMessage;
}
// Standard lifecycle hooks used to subscribe and unsubsubscribe to the message channel
connectedCallback() {
this.subscribeToMessageChannel();
}
disconnectedCallback() {
this.unsubscribeToMessageChannel();
}
}
Hi, this is a comment.
To get started with moderating, editing, and deleting comments, please visit the Comments screen in the dashboard.
Commenter avatars come from Gravatar.